Programming #
NORVI EC-M11-EG-C4 has a mini USB Port for serial connection with the SoC for programming. Any ESP32 supported programming IDE can be used to program the controller. Follow this Guide to programming NORVI ESP32 Based Controllers with Arduino IDE.
SoC: ESP32-WROOM32
Programming Port: USB UART
8-pin Connector and wire harness #
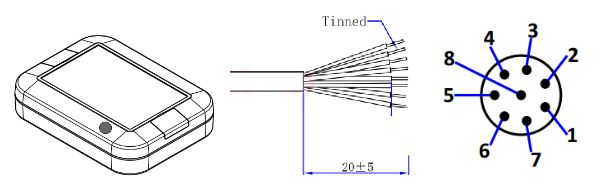
Pin Description #
8P Male | Wire color | I/O Configuration |
1 | White | Thermocouple + |
2 | Brown | Thermocouple – |
3 | Green | – |
4 | Yellow | – |
5 | Gray | – |
6 | Pink | – |
7 | Blue | Power+ |
8 | Red | Power- |
Thermocouple Input #
SPI MISO | GPIO19 |
SPI SCK | GPIO18 |
CS | GPIO5 |
Programming Thermocouple Inputs #
#include <SPI.h>
#include "Adafruit_MAX31855.h"
#define MAXDO 19
#define MAXCS 5
#define MAXCLK 18 // Initialize the Thermocouple
Adafruit_MAX31855 thermocouple(MAXCLK, MAXCS, MAXDO);
void setup() {
Serial.begin(115200);
Serial.println("MAX31855 test"); // Wait for MAX chip to stabilize
delay(500);
Serial.print("Initializing sensor...");
if (!thermocouple.begin()) {
Serial.println("ERROR.");
while (1) delay(10);
}
Serial.println("DONE.");
}
void loop() {
Serial.print("Internal Temp = ");
Serial.println(thermocouple.readInternal());
double c = thermocouple.readCelsius();
if (isnan(c)) {
Serial.println("Thermocouple fault(s) detected!");
uint8_t e = thermocouple.readError();
if (e & MAX31855_FAULT_OPEN) Serial.println("FAULT: Thermocouple is open - no connections.");
if (e & MAX31855_FAULT_SHORT_GND) Serial.println("FAULT: Thermocouple is short-circuited to GND.");
if (e & MAX31855_FAULT_SHORT_VCC) Serial.println("FAULT: Thermocouple is short-circuited to VCC.");
}
else {
Serial.print("C = ");
Serial.println(c);
}
//Serial.print("F = ");
//Serial.println(thermocouple.readFahrenheit());
Serial.println("");
Serial.print("Analog Read : ");
Serial.print(analogRead(36));
Serial.println("");
delay(1000);
}