Programming #
The NORVI AGENT 1-BM01-ES-L has a mini USB port for serial connection with the SoC for programming. Any ESP32-supported programming IDE can be used to program the controller. Follow this Guide to programming NORVI ESP32-based controllers with the Arduino IDE.
SoC: ESP32-WROVER-B
Programming Port: USB UART
Digital Inputs #
Wiring Digital Inputs #
The digital inputs of NORVI AGENT 1-BM01-ES-L can be configured as both sink and source connections. The inverse of the Digital Input polarity should be supplied to the common terminal.
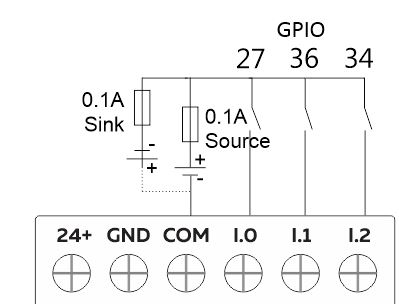
Programming Digital Inputs #
Reading the relevant GPIO of the ESP32 gives the value of the Digital Input. When the inputs are in the OFF state, the GPIO goes HIGH, and when the input is in the ON state, the GPIO goes LOW. Refer to the GPIO allocation table in the datasheet for the digital input GPIO.
#define INPUT1 27
void setup() {
Serial.begin(115200);
Serial.println("Device Starting");
pinMode(INPUT1, INPUT);
}
void loop() {
Serial.print(digitalRead(INPUT1));
Serial.println("");
delay(500);
}
0 – 10V Analog Input #
Wiring Analog Inputs #
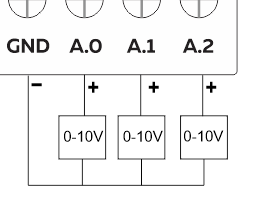
Reading Analog Input #
Reading the relevant I2C address of the ADC gives the value of the Analog Input.
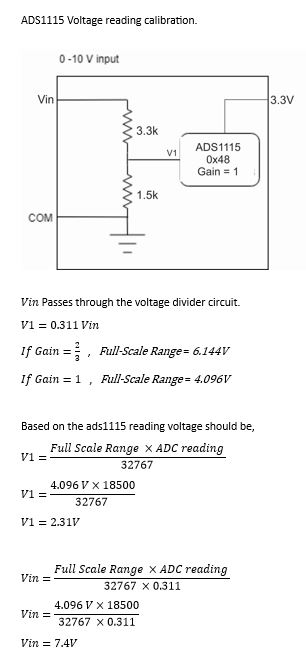
Programming Analog Inputs #
#include <Adafruit_ADS1X15.h>
#include <Wire.h>
Adafruit_ADS1115 ads1;
void setup() {
Serial.begin(115200);
Serial.println("Device Starting");
Wire.begin(21,22);
ads1.begin(0x48);
ads1.setGain(GAIN_ONE);
}
void loop() {
Serial.print("Analog 0 ");
Serial.println(ads1.readADC_SingleEnded(0));
delay(10);
Serial.print("Analog 1 ");
Serial.println(ads1.readADC_SingleEnded(1));
delay(10);
Serial.print("Analog 2 ");
Serial.println(ads1.readADC_SingleEnded(2));
delay(10);
Serial.println("");
delay(500);
}
RS-485 Communication #
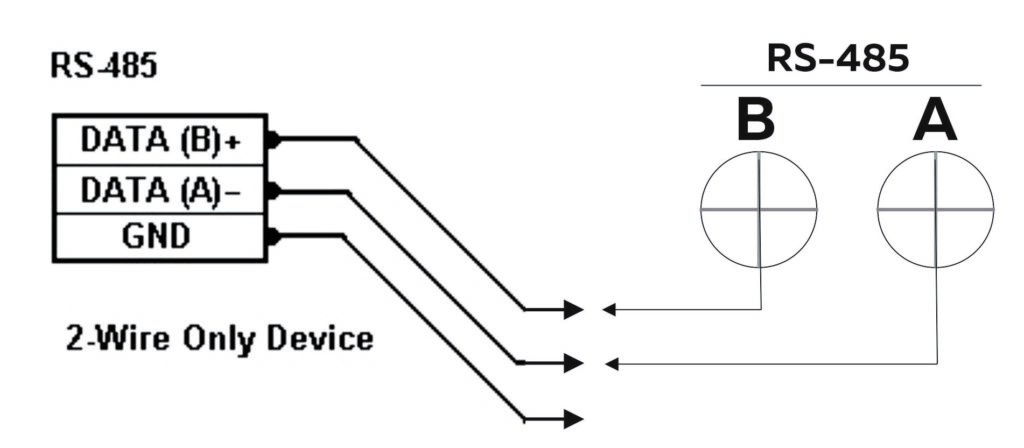
Driver | MAX485 |
UART RX | GPIO23 |
UART TX | GPIO13 |
Flow Control | GPIO32 |
Programming RS-485 #
The RS-485 connection of the NORVI AGENT 1-BM series uses
#define RXD 23
#define TXD 13
#define FC 32
void setup() {
Serial.begin(9600);
pinMode(FC, OUTPUT);
Serial1.begin(9600, SERIAL_8N1,RXD,TXD);
}
void loop() {
digitalWrite(FC, HIGH); // Make FLOW CONTROL pin HIGH
Serial1.println("RS485 01 SUCCESS"); // Send RS485 SUCCESS serially
delay(500); // Wait for transmission of data
digitalWrite(FC, LOW); // Receiving mode ON
while (Serial1.available()) { // Check if data is available
char c = Serial1.read(); // Read data from RS485
Serial.write(c); // Print data on serial monitor
}
delay(1000);
}
Built-in Buttons #
Button 1 Pin | Digital Input GPIO35 |
Programming Buttons #
#include <Adafruit_NeoPixel.h>
#define BUTTON_PIN 35 //Digital IO pin connected to the button. This will be
#define PIXEL_PIN 25 // Digital IO pin connected to the NeoPixels.
#define PIXEL_COUNT 1
Adafruit_NeoPixel strip = Adafruit_NeoPixel(PIXEL_COUNT, PIXEL_PIN, NEO_GRB + NEO_KHZ800);
bool oldState = HIGH;
int showType = 0;
void setup() {
Serial.begin(115200);
pinMode(BUTTON_PIN, INPUT_PULLUP);
strip.begin();
strip.show(); // Initialize all pixels to 'off'
Serial.println("RS-485 TEST");
}
void loop() {
bool newState = digitalRead(BUTTON_PIN);
if (newState == LOW && oldState == HIGH) {
delay(20);
newState = digitalRead(BUTTON_PIN);
if (newState == LOW) {
showType++;
if (showType > 9)
showType = 0;
startShow(showType);
}
}
oldState = newState;
}
void startShow(int i) {
switch (i) {
case 0: colorWipe(strip.Color(0, 0, 0), 50); break;
case 1: colorWipe(strip.Color(255, 0, 0), 50); break;
case 2: colorWipe(strip.Color(0, 255, 0), 50); break;
case 3: colorWipe(strip.Color(0, 0, 255), 50); break;
case 4: theaterChase(strip.Color(127, 127, 127), 50); break;
case 5: theaterChase(strip.Color(127, 0, 0), 50); break;
case 6: theaterChase(strip.Color(0, 0, 127), 50); break;
case 7: rainbow(20); break;
case 8: rainbowCycle(20); break;
case 9: theaterChaseRainbow(50); break;
}
}
LoRaWAN #
Specification | Long Range(LoRa) |
---|---|
RF Transceiver | RFM95W-915S2 |
bit Rate | Up to 300 kbps |
Link Budget | +20 dBm – 100 mW |
RF sensitivity | -148 dBm |
SPI MISO | GPIO19 |
SPI MOSI | GPIO23 |
SPI SCK | GPIO18 |
NSS | GPIO2 |
DIO0 | GPIO4 |
DIO1 | GPIO15 |
DIO2 | NOT CONNECTED |
RESET | GPIO5 |
USB and Reset #
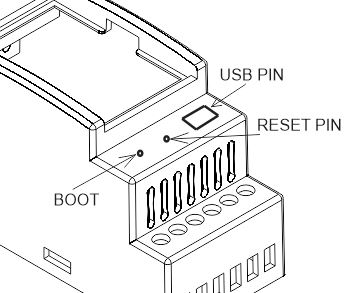