Programming #
The NORVI EC-M11-BC-C3 has a mini USB port for serial connection with the SoC for programming. Any ESP32-supported programming IDE can be used to program the controller. Follow this guide to programming NORVI ESP32-based controllers with the Arduino IDE.
SoC: ESP32-WROOM32
Programming Port: USB UART
Wiring Load Cell Input and Solar. #
8-pin and 3-pin connectors and wire harness #
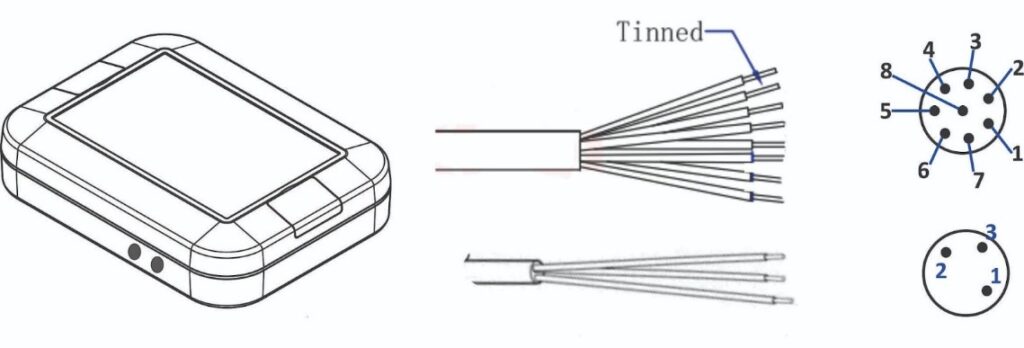
Pin Description #
8P Male | Wire color | I/O Configuration |
1 | White | A+ |
2 | Brown | A- |
3 | Green | B+ |
4 | Yellow | B- |
5 | Gray | – |
6 | Pink | – |
7 | Blue | – |
8 | Red | – |
3P Male | Wire color | I/O Configuration |
1 | Blue | Solar Panel + |
2 | Black | Not in Use |
3 | Brown | Solar Panel – |
Load Cell Inputs #
Programming Load Cell Inputs #
Number of Load Cell Inputs | 1 |
Module Type | HX711 |
PD SCK | GPIO12 |
DOUT | GPIO13 |
#include "HX711.h"
const int LOADCELL_DOUT_PIN = 13;
const int LOADCELL_SCK_PIN = 12;
HX711 scale;
void setup() {
Serial.begin(115200);
Serial.println("HX711 Demo");
Serial.println("Initializing the scale");
scale.begin(LOADCELL_DOUT_PIN, LOADCELL_SCK_PIN);
scale.set_scale(2280.f);
// this value is obtained by calibrating the scale with known weights;
scale.tare(); // reset the scale to 0
Serial.println("After setting up the scale:");
Serial.print("read: \t\t");
Serial.println(scale.read()); // print a raw reading from the ADC
Serial.print("read average: \t\t");
Serial.println(scale.read_average(20));
// print the average of 20 readings from the ADC
Serial.print("get value: \t\t");
Serial.println(scale.get_value(5));
// print the average of 5 readings from the ADC minus the tare weight,
//set with tare()
Serial.print("get units: \t\t");
Serial.println(scale.get_units(5), 1);
// print the average of 5 readings from the ADC minus tare weight, divided
Serial.println("Readings:");
}
void loop() {
Serial.print("one reading:\t");
Serial.print(scale.get_units(), 1);
Serial.print("\t| average:\t");
Serial.println(scale.get_units(10), 1);
}
Solar Input #
Solar Powered Model | CN3083 |
Maximum Charge Current | 600mA |
Maximum Voltage | 6V |
Input Voltage monitor | ADS1115 – 0x49 – AIN2 |
Battery Input #
Battery Type | 103040 Lithium polymer battery |
Nominal Capacity | 1200mAh |
Nominal Voltage | 3.75V |
Overcharge | 4.2V |
Over-discharge Cutoff Voltage | 3V |
Programming Solar and Battery #
#include <Adafruit_SSD1306.h>
#include <Adafruit_ADS1X15.h>
Adafruit_ADS1115 ads1;
int analog_value = 0;
void setup() {
Serial.begin(115200);// put your setup code here, to run once:
Wire.begin(16,17);
if (!ads1.begin(0x49)) {
Serial.println("Failed to initialize ADS 1 .");
while (1);
}
}
void loop() {
int16_t adc0, adc1, adc2, adc3;
adc0 = ads1.readADC_SingleEnded(0);
adc1 = ads1.readADC_SingleEnded(1);
adc2 = ads1.readADC_SingleEnded(2);
adc3 = ads1.readADC_SingleEnded(3);
Serial.println("-----------------------------------------------------------");
Serial.print("AIN1: ");
Serial.print(adc0);
Serial.println(" ");
Serial.print("AIN2: ");
Serial.print(adc1);
Serial.println(" ");
Serial.print("SOLAR: ");
Serial.print(adc2);
Serial.println(" ");
Serial.print("AIN4: ");
Serial.print(adc3);
Serial.println(" ");
}